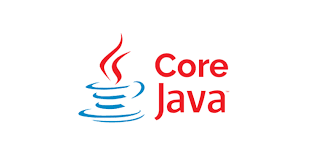
CORE JAVA
JAVA PROGRAMMING
1: Introduction
- What is Java.
- How to Install & set Path.
- A Simple Java Program
- Compiling & executing Java Program
- Phases of Java Program
- Analysis of a Java Program
- Name of a Java Source File
2: Fundamentals of Java Programming
- Naming convention of Java language
- Comments
- Statements
- Blocks (Static, Non-static/instance)
- Identifiers
- Keywords
- Literals
- Primitive Data Types, Range
- Reference (User defined) Data type
- Variables (Primitive, Reference)
- Type Casting, Default Value
- Operators
3: Control Structures
- Working with Control Structures
- Types of Control Structures
- Decision Control Structure (if, if-else, if else if, switch –case)
- Repetition Control Structure (do –while,while, for)
- Program/Interview questions
4: Input Fundamentals and Datatypes In Java
- Java program inputs from Keyboard
- Methods of Keyboard inputs
- Scanner
- Java Array
- What is Array
- Instantiation of an Array
- Elements, Default Value, for-each loop, varargs
- Length of an Array (What is –ArrayIndexOutOfBoundsException)
- Increasing, Decreasing the Size and Copy of an Array
- Multi-Dimensional Arrays
5: Command-Line Arguments
- What is a Command-Line Argument?
- Java Application with Command-Line Arguments
- Conversion of Command-Line Arguments
- Passing Command-Line Arguments
- Using Eclipse IDE
6: Object Oriented Programming
- Procedural Vs Object Oriented Program
- Different type of Program Procedural Vs Object Oriented.
- Top Down Vs Bottom Up Approach
- Introduction to Object Oriented
- Abstraction, Encapsulation, Inheritance,
- Polymorphism.
7: Object Oriented Programming
- Introduction to Classes and Objects
- Custom Class Definition
- Instance and Static Variables
- Instance Variable and it’s role in a Class
8: Constructor and Static
- Constructors, types of Constructor,
- Constructor Rule, Constructor Overloading
- Static Variable and it’s use.
- Methods(Static & Non static) and their behaviour.
- Using blocks (Static, Non Static)
- Constructor vs Methods
- “this” Keyword
- Java Access Modifiers (and Specifiers)
- Call by value, Call by reference
- Different ways to create Object Instance
9: Inner Class
- First View of Inner Class
- Outer Class Access
- Types of Inner Class
10: Inheritance
- Complete concepts of Inheritance
- Sub-Classes
- Object Classes
- Constructor Calling Chain
- The use of “super” Keyword
- The use of “private” keyword inheritance.
- Reference Casting
11: Abstract Classes and Inheritance
- Introduction to Abstract Methods
- Abstract Classes and Interface
- Interface as a Type
- Interface v/s Abstract Class
- Interface Definition
- Interface Implementation
- Multiple Interfaces’ Implementation
- Interfaces’ Inheritance
- How to create object of Interface
12: Polymorphism
- Introduction to Polymorphism
- Types of Polymorphism
- Polymorphic Behavior in Java
- Benefits of Polymorphism
- Overloading Methods
- Covariant return type in Overriding Methods
- Hiding Methods
- Final Class and Method
- “Is-A” vs “Has-A”
- Association Vs Aggregation
13:Package
- Package and Class path and its use
- First look into Packages
- Benefits of Packages
- Package Creation and Use
- First look into Class path
- Class path Setting
- Class Import
- Package Import
- Role of public, protected, default and private w.r.t package
- Namespace Management
- Package vs. Header File
- Creating and Using the Sub Package
14:Using Predefined Package & Other Classes
- Java.lang Hierarchy
- Object class and using toString(), equals(),hashCode(), clone(), finalize() etc
- Using Runtime Class, Process Class to play music, video from Java Program
- Primitives and Wrapper Class
- Math Class
- Wrapper Classes
- System Class using gc(), exit(), etc.
15: String Handling
- String, StringBuffer, StringBuilder Class
- String Constant Pool
- Various usage and methods of String,StringBuffer, StringBuilder
16: New Concepts In Package
- Auto boxing and Auto unboxing
- Static import.
- Instance of operator.
- Enum and its use in Java
- Working with jar
- Garbage Collection Introduction
- Advantages of Garbage Collection
- Garbage Collection Procedure
- Java API
17: Exception Handling
- Introduction to Exceptions
- Effects of Exceptions
- Exception Handling Mechanism
- Try, catch, finally blocks
- Rules of Exception Handling
- Exception class Hierarchy, Checked &
- Unchecked Exception
- Throw & throws keyword
- Custom Exception Class
- Chained Exception.
- Resource handling & multiple exception class
18: Multithreading
- Introduction
- Advantages
- Creating a Thread by inheriting from Thread class
- Run() and start() method.
- Constructor of Thread Class
- Various Method of Thread Class
- Runnable Interface Implementation
- Thread Group
- Thread States and Priorities
- Synchronization method, block
- Class & Object Level Lock
- Deadlock & its Prevention
- Inter thread Synchronization
- Life Cycle of Thread
- Deprecated methods : stop(), suspend(),resume(), etc
19: Input and Output Streams
- Java I/O Stream
- I/O Stream – Introduction
- Types of Streams
- Stream Class Hierarchy
- Using File Class
- Copy and Paste the content of a file
- Byte Streams vs Character Streams
- Text File vs Binary File
- Character Reading from Keyboard by Input Stream Reader
- Reading a Line/String from Keyboard by Buffered Reader
- Standard I/O Streams Using Data Streams to read/write
- primitive data
- PrintStream vs PrintWriter Using StreamTokenizer and RandomAccessFile
- Introduction to Serialization
- Using Object Streams to read/write object
- Transient Keyword
- Serialization Process
- Deserialization Process
20: Socket Programming
- Introduction to Java Network Programming,
- Using InetAddress, Socket, and ServerSocket
- Using suitable data streams & data transfer
- Creating Socket Application Using TCP/IP
- Creating Socket Application Using UDP
- Developing multi threaded server for handling concurrent clients
- Using URLConnection class
21: Collection Framework
- Generics(Templates)
- What is generic
- Creating User defined Generic classes
- The java.util package
- Collection
- What is Collection Framework
- Legacy and non-legacy collection classes
- List, Set & Map interfaces
- Using Vector, Array List, Stack,
- Using Collections class for sorting
- Linked List.
- Using HashSet, TreeSet, LinkedHashSet etc
- Using Hashtable, Hash Map, Tree Map,
- Iterator, Enumerator.
- SortedMap, LinkedHashMap etc.
- Using Queue, Deque, SortedQue, etc.
- Using Random class
- Using user defined class for DataStructure
- Using Date and Formatting Date class.
- Using Properties in a Java Program
- Reflection Overview,Annotation Overview
22: JDBC
- What is JDBC, Driver architecture,The java.sql package
- Using DriverManager, Connection, Statement
- Using ResultSet to fetch data, how to create Scrollable and Updatable ResultSet
- Using PreparedStatement to manipulate DB
- Stored procedure & functions,CallableStatement
- Handling BLOB to store & retrieve binary content
Session 23: GUI
- What is Applet
- How to create a frame
- AWT Components
- Layout Managers
- What is Event
- Event handling
- Event handling
24: JDBC (Java Database connectivity )
Introduction
Connecting with Data base
CURD Operations
25.Project
Trainer
Prof Gitesh Mallick
Mrp Price
₹ 8000
Discount In Percentage
13% off
Discounted Fee
₹ 7000
Duration
80 Hr